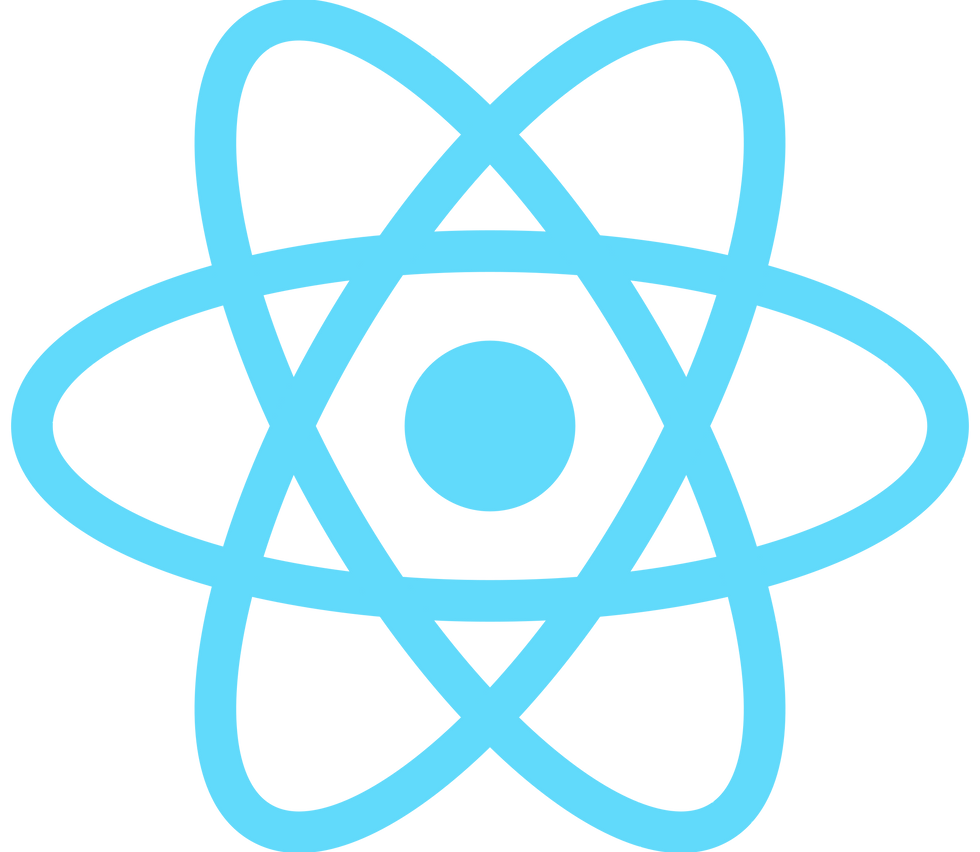
Hello fellow developers and blog warriors. I would like to begin by thanking you all for the amazing, and constructive feedback on my last blog post regarding “Learning REACT - App Development Simplified”. It was great to know that there are others out there that found value in approach to learning new technologies.
For my second post regarding app development in React, we will dive deeper and explore some intermediate to advanced topics. The goal is to learn various techniques for creating dynamic web pages. We will also build an exciting Idea Tracker project! So buckle up… this one may cause some turbulence, or just send you straight into the ditch. Kidding! I will try my best to break down each section as well as provide visual examples. If at any point you have any questions or need further clarification please ask them in the comments below. With that being said, let's dig into it!
Static Pages
The "Hello, World!" application is a staple of programming culture. It's the first thing you learn to build when you're starting in a new programming language. This program is super simple at its core. To create this application in React, you can use the ReactDOM.render() method to render a React component into a container element in your HTML file. Or if you already have your react project setup, you can add your text inside an <h1> or <p> tag inside your JSX component and voila, you have yourself a static “Hello, World!” web application.
VS Code
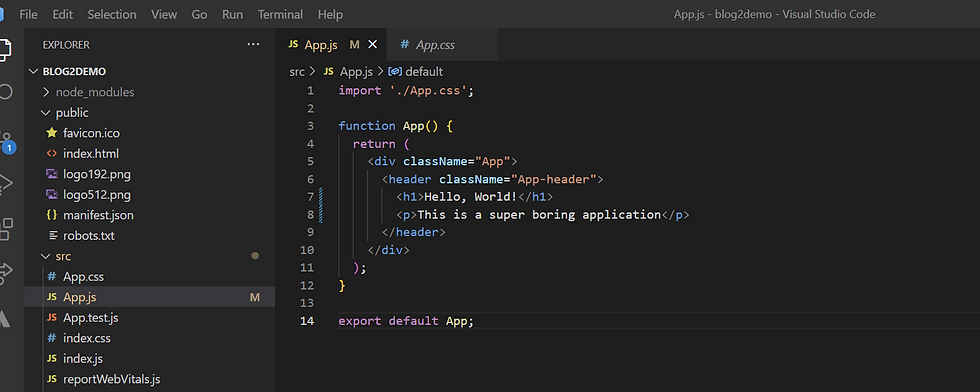
Output: localhost 3000:

Now you could do that…why would you? In React we want to build dynamic web pages that users can interact with. Modern applications use forms, buttons, drop down menus, sliders etc to hook your attention. This is what makes apps so functional, and addicting. There are plenty of tools within JS and React that allow you to build engaging and useful applications. In the next section we will explore these tools in depth by creating a project!
Dynamic Pages:
Dynamic web pages are an exciting way to create engaging and interactive experiences for users. It makes the browsing experience more immersive and enjoyable. With dynamic pages, I can easily change the content on the fly, without having to refresh the entire page. This allows for a more seamless and efficient browsing experience.
Additionally, dynamic web pages can be customized to meet the specific needs and preferences of different users. For example, an e-commerce site can use dynamic pages to show personalized product recommendations based on a user's browsing and purchasing history. This helps to create a more tailored shopping experience and increases the chances of a sale. The switch from static to dynamic web pages was important enough to define the current era of the modern web: “Web 2.0”.
As important as dynamic applications are, building them is easier said than done. Web pages that respond to user actions in real-time can be a bit tricky and require a much deeper “bag of tricks''.
In order to get started building dynamic web pages, you will need a comprehensive understanding of the following topics:
Components:
In the last blog post we went over components. I only bring this up again because of how important it is. React components are the elementary building blocks of all React applications. You should know how to create functional and class components, how to use props to pass data between components, and how to use state to manage component state. Components usually import react from ‘react’, start with a function, have a return statement as well as an export statement.
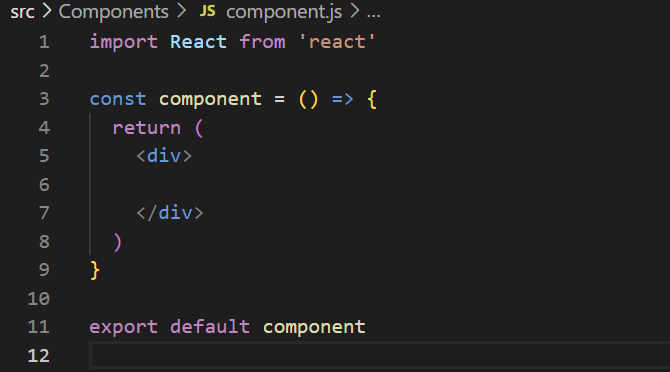
JSX:
As previously discussed in the last blog post, JSX allows you to write HTML-like code in your JavaScript files. You should be comfortable with using JSX to create and render React components.
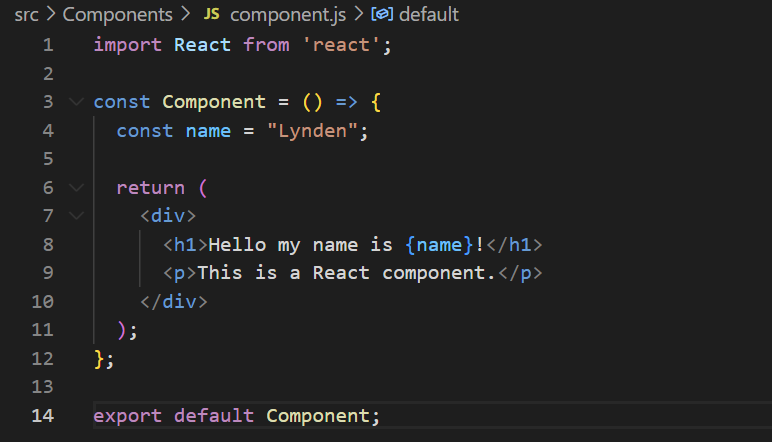
Hooks:
Hooks important features in React that let developers expand the capabilities of their application. Before hooks, you could only use these things in class components, which could be kind of annoying to set up. Introduced in version 16.8, hooks can make your code simpler and more readable, and make your life as a React developer a lot easier!
useState: One of the most common hooks. This allows a component to manage its own state. With useState, you can create stateful values that can be updated and accessed by the component.
useEffect: This hook allows a component to perform side effects, such as fetching data or subscribing to events, after rendering. useEffect can be used to keep the component in sync with external data sources.
useContext: This hook allows a component to access and use values from a React context. Context is a way to pass data down the component tree without having to manually pass props at each level.
useReducer: This hook is an alternative to useState that allows for more complex state management. It's similar to the reducer pattern used in Redux.
useMemo: This hook allows a component to memoize expensive computations so they are not re-executed on every render. Memoization can improve performance by avoiding unnecessary re-renders.
useCallback: This hook allows a component to memoize event handlers and other functions to avoid unnecessary re-renders. useCallback can be used to optimize performance in components that rely heavily on event handlers.
useRef: This hook allows a component to create and maintain a mutable reference to a DOM element or other value. useRef can be used to store references to elements that need to be manipulated directly, or to store any other kind of mutable value.
Examples of Hooks:
For this blog I will only provide two examples of the most commonly used hooks. You can find more information on hooks here: https://legacy.reactjs.org/docs/hooks-overview.html
useState()
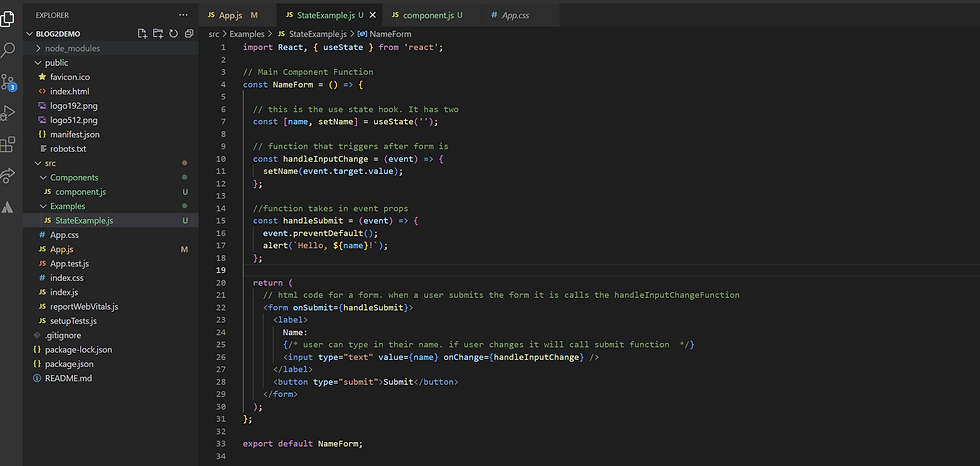
useEffect()
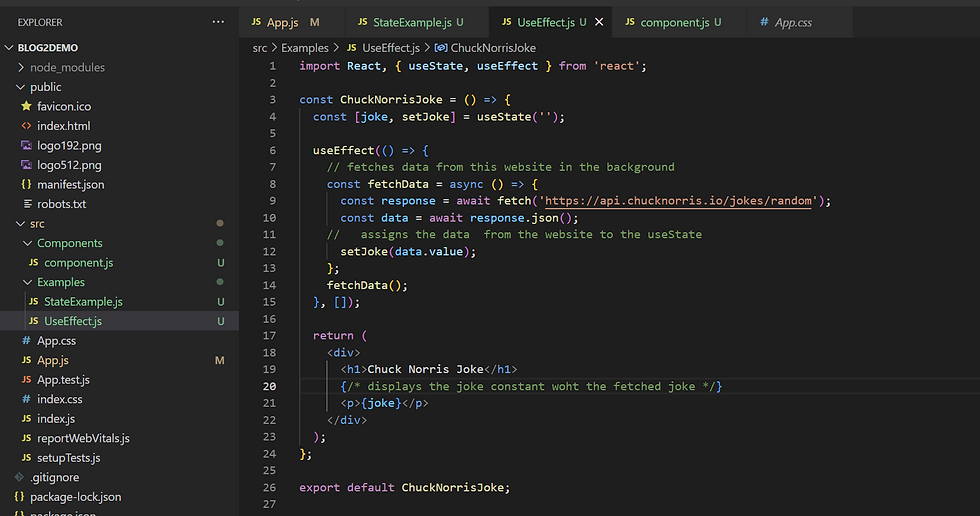
Event Handling:
handling user events such as clicking, hovering, or typing is super important. In this example we will combine the useState hook with an onClick handler to increment the count of the button every time it is pressed.
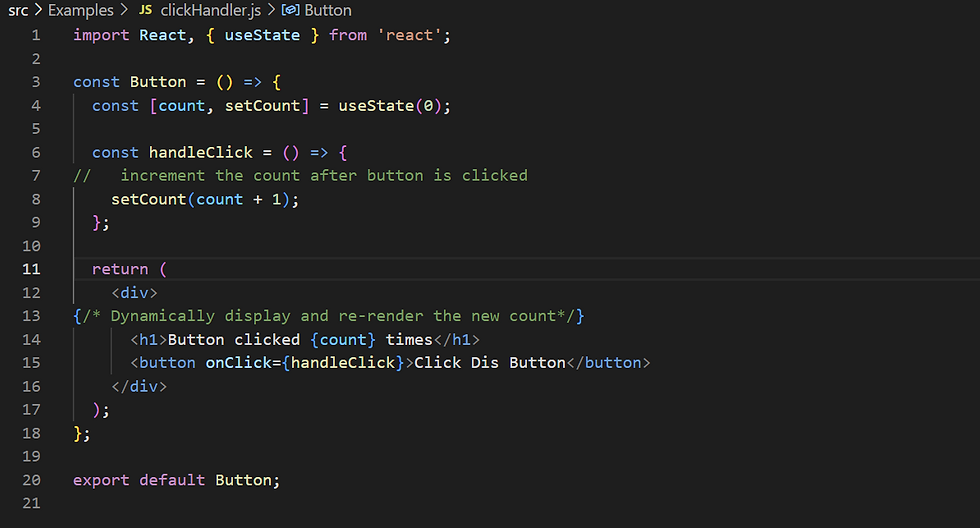
Routing:
Routing is only important if you are building multi page applications or jumping between components. Managing routing in a React application will help you navigate between different components or pages based on the URL. This is useful if you are building large applications. We won’t use it for our project but it is super useful to know and understand.
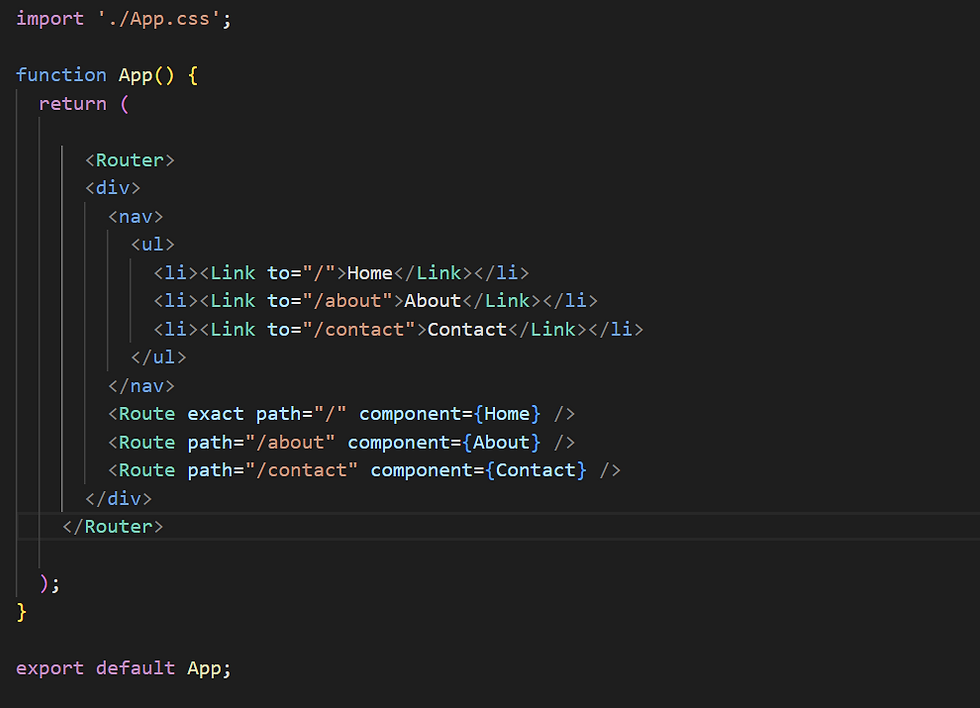
The Next Step: Converting Knowledge to Wisdom
Learning is an endless journey that I approach with a thirst for knowledge. I consume a variety of materials, from youtube videos to textbooks, but I've learned that simply absorbing information isn't enough. To truly understand a subject, I must put my learnings into practice.
I liken the process of learning to storing information in RAM (random access memory) in a computer. It's volatile and easily forgotten, so I aim to move that information into long-term storage by applying it to real-world projects. This involves converting data into meaningful information, storing it as knowledge, and recalling it as wisdom.
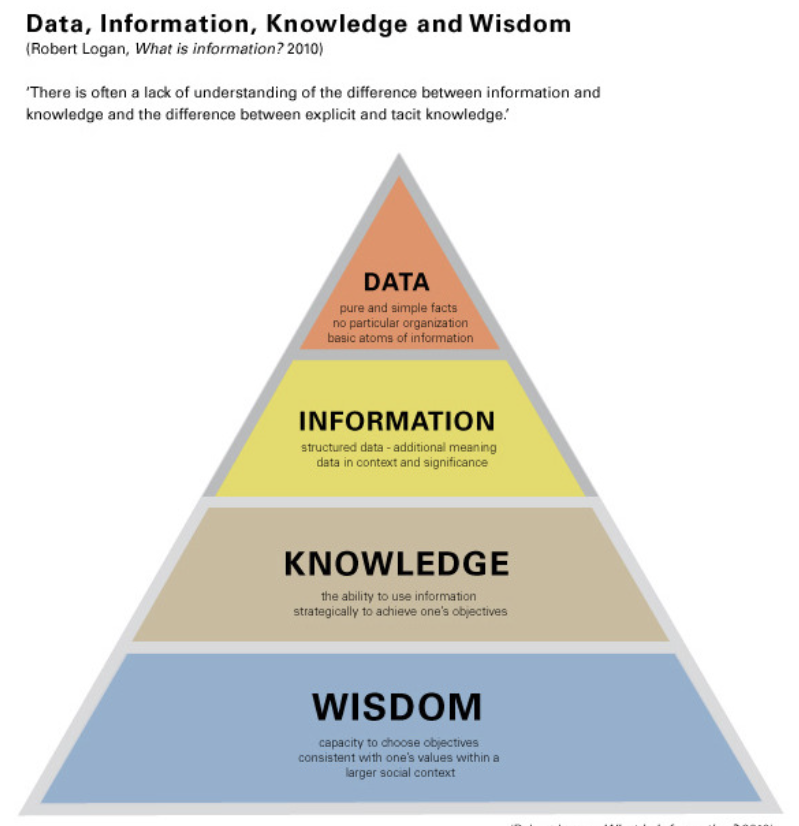
Project
Today, we'll be taking our knowledge to the next level by creating an Idea Tracker Application. It's a spin on the classic To-Do List tracker, but it has the potential to benefit me in my creative endeavors. By following along, you'll not only enhance your programming skills, but also gain a valuable portfolio piece that could impress potential employers in the software development field. So, let's get started on this exciting journey of converting our knowledge into wisdom.
Installation
Step 1: Create-React-App
Step 2: Instal u uid - to generate unique id’. In a new terminal run this command
npm install uuid
Step 3 install font awesome. In a new terminal run this command
npm install --save @fortawesome/fontawesome-free
Step 4: Run npm start to launch the application for the first time. Make sure you get rid of the react Icon.
Project Set Up
Step 5: Create a folder called Components
Step 6: Inside the components folder create 4 js files.
EditTodoForm.js
Todo.js
TodoForm.js
TodoWrapper.js
Step 7: remove everything from your app.js so that the main component returns only two <div> tags. Then Import your todoWrapper component and use it within the div as JSX. also give a className to the top <div>.
import "./App.css";
import TodoWrapper from "./Components/TodoWrapper";
function App() {
return (
<div className="App">
<TodoWrapper />
</div>
);
}
export default App;
Step 8: Grab the CSS from the src/Examples/App.css and copy and paste it into a new file called App.css. Feel free to customize the colours fonts and divs to your liking!
Step 9: inside your ToDoForm.js and type ‘rafce’ to create a standard export component that matches the filename.
Insert or type out this code to create a form with a button:
import React from "react";
const TodoForm = () => {
return (
<form className="TodoForm">
<input
type="text"
className="todo-input"
placeholder=" What ideas do you have today?"
></input>
<button type="submit" className="todo-btn"></button>
</form>
);
};
export default TodoForm;
Step 10: inside TodoWrapper we first want to import the ToDoForm component. Create a Div and assign it a className for CSS styling. Within the Div add your TodoForm Component
import React from "react";
import TodoForm from "./TodoForm";
const TodoWrapper = () => {
return (
<div className="TodoWrapper">
<TodoForm />
</div>
);
};
export default TodoWrapper;
The application should now appear like this:
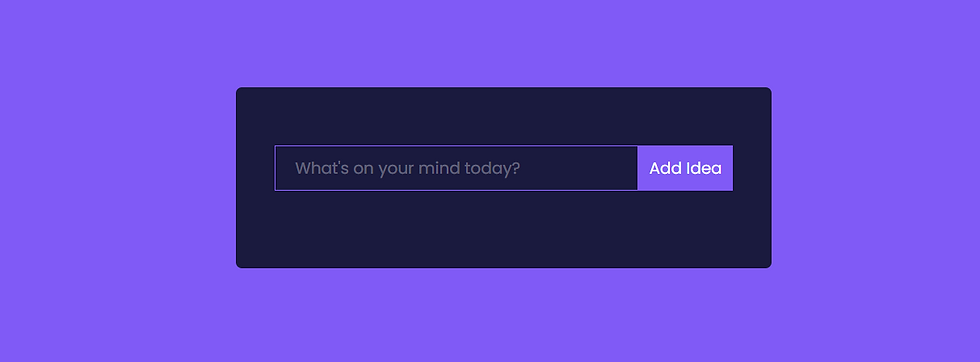
Step 11: Next we need to keep track of what the user is typing in. So we need to use the useState hook that we learned about earlier. Navigate back to the TodoForm.js file and add useState in the import statement. Next, go right below the const function and add the useState.
const TodoForm = () => {
const [value, setvalue] = useState("")
return (
We will then go to our form and add an onChange handler. We can test the handler by console logging the value of each keystroke. Go to your app then open dev tools and check to make sure the console is logging what you type.
This code was added to the input element:
onChange={(e) => console.log(e.target.value)}
Now you just need to change the console.log and instead assign it to ‘setValue’ for the useState.
onChange={(e) => setvalue(e.target.value)}
Step 12: Next we need to add an onSubmit handler to the form so that it captures and handles the current state (captures the typed idea).
<form className="TodoForm" onSubmit= {handleSubmit}>
But we also need to add a handler for it. For that we can create a new function called handleSubmit which will take an event. We also need to prevent the page from reloading by default by adding this:
const handleSubmit = e => {
e.preventDefault();
console.log(value)
}
We console log it just to make sure that when the submit button is clicked it returns the full idea query. Now your TodoForm.js page should look something like this
import React, {useState} from "react";
const TodoForm = () => {
const [value, setvalue] = useState("")
const handleSubmit = e => {
e.preventDefault();
console.log(value)
}
return (
<form className="TodoForm" onSubmit= {handleSubmit}>
<input
type="text"
className="todo-input"
placeholder="What's on your mind today?"
onChange={(e) => setvalue(e.target.value)}
></input>
<button type="submit" className="todo-btn">
Add Idea
</button>
</form>
);
};
export default TodoForm;
Thank you for joining me in today's tutorial! I hope you found it informative and engaging, and that you feel confident about getting started with building dynamic web pages. If you have any questions or feedback, please don't hesitate to leave them in the comment section below. Your input is greatly appreciated and I am always here to help you in your web development journey. Thanks again for your time and attention, and happy coding!
Comments